Loading and Animating the Panda Model
Actors
The Actor
class is for animated models. Note
that we use loadModel()
for static
models and Actor
only when they are animated.
The two constructor arguments for the Actor
class are the name of the file containing the model and a Python dictionary
containing the names of the files containing the animations.
Update the Code
1from math import pi, sin, cos
2
3from direct.showbase.ShowBase import ShowBase
4from direct.task import Task
5from direct.actor.Actor import Actor
6
7
8class MyApp(ShowBase):
9 def __init__(self):
10 ShowBase.__init__(self)
11
12 # Load the environment model.
13 self.scene = self.loader.loadModel("models/environment")
14 # Reparent the model to render.
15 self.scene.reparentTo(self.render)
16 # Apply scale and position transforms on the model.
17 self.scene.setScale(0.25, 0.25, 0.25)
18 self.scene.setPos(-8, 42, 0)
19
20 # Add the spinCameraTask procedure to the task manager.
21 self.taskMgr.add(self.spinCameraTask, "SpinCameraTask")
22
23 # Load and transform the panda actor.
24 self.pandaActor = Actor("models/panda-model",
25 {"walk": "models/panda-walk4"})
26 self.pandaActor.setScale(0.005, 0.005, 0.005)
27 self.pandaActor.reparentTo(self.render)
28 # Loop its animation.
29 self.pandaActor.loop("walk")
30
31 # Define a procedure to move the camera.
32 def spinCameraTask(self, task):
33 angleDegrees = task.time * 6.0
34 angleRadians = angleDegrees * (pi / 180.0)
35 self.camera.setPos(20 * sin(angleRadians), -20 * cos(angleRadians), 3)
36 self.camera.setHpr(angleDegrees, 0, 0)
37 return Task.cont
38
39
40app = MyApp()
41app.run()
The command loop("walk")
causes
the walk animation to begin looping.
Run the Program
The result is a panda walking in place as if on a treadmill:
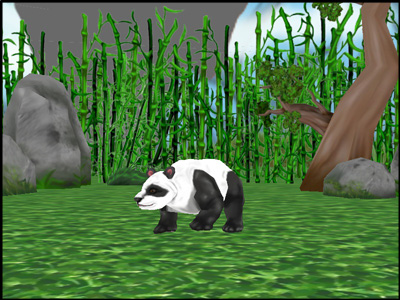