Loading and Animating the Panda Model
Update the Code
The Actor
class which is available to Python
users is not available to C++ users. you should create your own Actor class
which at least should do the following:
load the Actor Model
load the animations
bind the model and the animations using AnimControl or AnimControlCollection
The next sample will load the panda model and the walk animation. the call:
window->loop_animations(0);
does the magic of binding all the loaded
models and their animations under the node path: render . it’s very important
to note that any animations loaded after the above call will not show until
the same method is called again. also any animations loaded under a node path
which doesn’t belong to render (for example: render_2d) will not show even if
the call: window->loop_animations(0);
is made. For such animations to
show, other steps must be applied (more on this later).
1#include "pandaFramework.h"
2#include "pandaSystem.h"
3
4#include "genericAsyncTask.h"
5#include "asyncTaskManager.h"
6
7// Global stuff
8PT(AsyncTaskManager) taskMgr = AsyncTaskManager::get_global_ptr();
9PT(ClockObject) globalClock = ClockObject::get_global_clock();
10NodePath camera;
11
12// Task to move the camera
13AsyncTask::DoneStatus SpinCameraTask(GenericAsyncTask *task, void *data) {
14 double time = globalClock->get_real_time();
15 double angledegrees = time * 6.0;
16 double angleradians = angledegrees * (3.14 / 180.0);
17 camera.set_pos(20 * sin(angleradians), -20.0 * cos(angleradians), 3);
18 camera.set_hpr(angledegrees, 0, 0);
19
20 return AsyncTask::DS_cont;
21}
22
23int main(int argc, char *argv[]) {
24 // Open a new window framework and set the title
25 PandaFramework framework;
26 framework.open_framework(argc, argv);
27 framework.set_window_title("My Panda3D Window");
28
29 // Open the window
30 WindowFramework *window = framework.open_window();
31 camera = window->get_camera_group(); // Get the camera and store it
32
33 // Load the environment model
34 NodePath scene = window->load_model(framework.get_models(), "models/environment");
35 scene.reparent_to(window->get_render());
36 scene.set_scale(0.25, 0.25, 0.25);
37 scene.set_pos(-8, 42, 0);
38
39 // Load our panda
40 NodePath pandaActor = window->load_model(framework.get_models(), "models/panda-model");
41 pandaActor.set_scale(0.005);
42 pandaActor.reparent_to(window->get_render());
43
44 // Load the walk animation
45 window->load_model(pandaActor, "models/panda-walk4");
46 window->loop_animations(0); // bind models and animations
47 //set animations to loop
48
49 // Add our task do the main loop, then rest in peace.
50 taskMgr->add(new GenericAsyncTask("Spins the camera", &SpinCameraTask, nullptr));
51 framework.main_loop();
52 framework.close_framework();
53 return 0;
54}
We are first loading the model file and the animation file like ordinary
models. Then, we are simply calling loop_animations(0)
to loop all
animations.
Run the Program
The result is a panda walking in place as if on a treadmill:
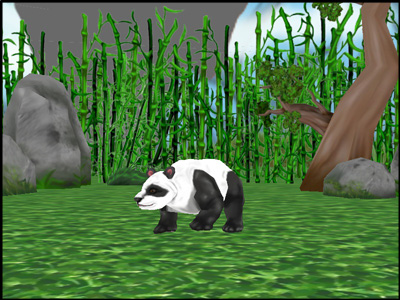