Loading and Animating the Panda Model
Update the Code
The Actor
class which is available to Python
users is not available to C++ users. you should create your own Actor class
which at least should do the following:
load the Actor Model
load the animations
bind the model and the animations using AnimControl or AnimControlCollection
The next sample will load the panda model and the walk animation. the call:
window->loop_animations(0);
does the magic of binding all the loaded
models and their animations under the node path: render . it’s very important
to note that any animations loaded after the above call will not show until
the same method is called again. also any animations loaded under a node path
which doesn’t belong to render (for example: render_2d) will not show even if
the call: window->loop_animations(0);
is made. For such animations to
show, other steps must be applied (more on this later).
1#include "pandaFramework.h"
2#include "pandaSystem.h"
3
4int main(int argc, char *argv[]) {
5 // Open a new window framework and set the title
6 PandaFramework framework;
7 framework.open_framework(argc, argv);
8 framework.set_window_title("My Panda3D Window");
9
10 // Open the window
11 WindowFramework *window = framework.open_window();
12 NodePath camera = window->get_camera_group(); // Get the camera and store it
13
14 // Load the environment model
15 NodePath scene = window->load_model(framework.get_models(), "models/environment");
16 scene.reparent_to(window->get_render());
17 scene.set_scale(0.25, 0.25, 0.25);
18 scene.set_pos(-8, 42, 0);
19
20 // Load our panda
21 NodePath panda = window->load_model(framework.get_models(), "models/panda-model");
22 panda.set_scale(0.005);
23 panda.reparent_to(window->get_render());
24
25 // Load the walk animation
26 window->load_model(panda, "models/panda-walk4");
27 window->loop_animations(0); // bind models and animations
28 //set animations to loop
29
30 // Add our task, which can be any function or lambda that returns DoneStatus.
31 framework.get_task_mgr().add("SpinCameraTask", [=](AsyncTask *task) mutable {
32 // Calculate the new position and orientation (inefficient - change me!)
33 double angledegrees = task->get_elapsed_time() * 6.0;
34 double angleradians = angledegrees * (3.14 / 180.0);
35 camera.set_pos(20 * sin(angleradians), -20.0 * cos(angleradians), 3);
36 camera.set_hpr(angledegrees, 0, 0);
37
38 // Tell the task manager to continue this task the next frame.
39 return AsyncTask::DS_cont;
40 });
41
42 // Run Panda's main loop until the user closes the window.
43 framework.main_loop();
44 return 0;
45}
We are first loading the model file and the animation file like ordinary
models. Then, we are simply calling loop_animations(0)
to loop all
animations.
Run the Program
The result is a panda walking in place as if on a treadmill:
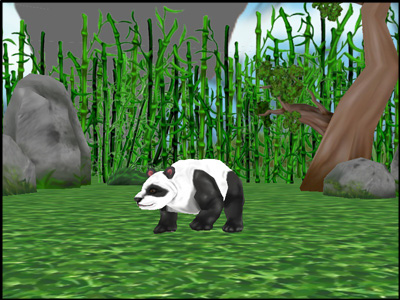