Running your Program
This section will explain how to compile a C++ program that uses the Panda3D libraries. On Windows, this is done using the Microsoft Visual C++ compiler; on all other systems, this can be done with either the Clang or the GCC compiler. Choose the section that is appropriate for your operating system.
Compiling your Program with Visual Studio
The descriptions below assume Microsoft Visual Studio 2015, but they should also work for 2017, 2019 and 2022.
Setting up the project
When creating a new project in Visual Studio, be sure to select the template for a “Win32 Console application” under the Visual C++ templates category. We recommend disabling “Precompiled headers” for now. (Don’t worry, you can still change these things later.)
When you created your project, the first thing you’ll need to do is change “Debug” to “Release” below the menu bar, as shown in the image below. This is because the SDK builds of Panda3D are compiled in Release mode as well. The program will crash mysteriously if the setting doesn’t match up with the setting that was used to compile Panda3D. This goes for the adjacent platform selector as well; select “x64” if you use the 64-bit Panda3D SDK, and “x86” if you use the 32-bit version.
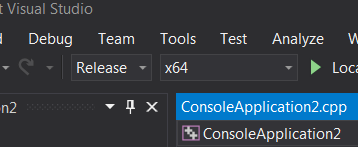
Now, open up the project configuration pages. Change the “Platform Toolset” in the “General” tab to “v140”.
Furthermore, we need to go to C/C++ -> “Preprocessor Definitions” and remove
the NDEBUG
symbol from the preprocessor definitions. This was
automatically added when we switched to “Release” mode, but having this
setting checked removes important debugging checks that we still want to keep
until we are ready to publish the application.
Now we are ready to add the paths to the Panda3D directories. Add the following paths to the appropriate locations (replace the path to Panda3D with the directory you installed Panda3D into, of course):
Include Directories
C:\Panda3D-1.11.0-x64\include
Library Directories
C:\Panda3D-1.11.0-x64\lib
Then, you need to add the appropriate Panda3D libraries to the list of “Additional Dependencies” your project should be linked with. The exact set to use varies again depending on which features of Panda3D are used. This list is a reasonable default set:
libp3framework.lib
libpanda.lib
libpandaexpress.lib
libp3dtool.lib
libp3dtoolconfig.lib
libp3direct.lib
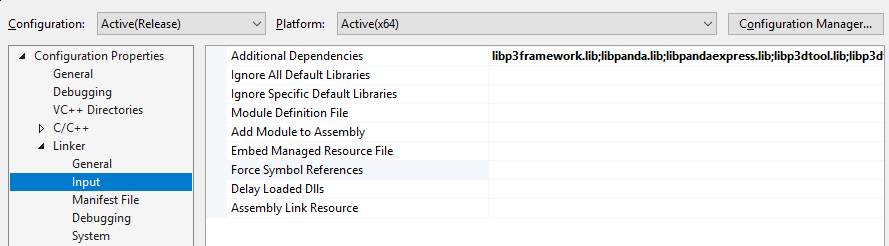
This should be enough to at least build the project. Press F7 to build your project and start the compilation process. You may see several C4267 warnings; these are harmless, and you may suppress them in your project settings.
There is one more step that needs to be done in order to run the project, though. We need to tell Windows where to find the Panda3D DLLs when we run the project from Visual Studio. Go back to the project configuration, and under “Debugging”, open the “Environment” option. Add the following setting, once again adjusting for your specific Panda3D installation directory:
PATH=C:\Panda3D-1.11.0-x64\bin;%PATH%
Now, assuming that the project built successfully, you can press F5 to run the program. Of course, not much will happen yet, because we don’t have any particularly interesting code added. The following tutorial will describe the code that should be added to open a Panda3D window and start rendering objects.
Compiling your Program with GCC or Clang
On platforms other than Windows, we use the GNU compiler or a compatible
alternative like Clang. Most Linux distributions ship with GCC out of the
box; some provide an easily installable package such as build-essential
on Ubuntu or the XCode Command-Line Tools on macOS. To obtain the latter, you
may need to register for an account on the
Apple developer site.
Having these two components, we can proceed to compile. The first step is to
create an .o file from our .cxx file. We need to specify the location of the
Panda3D include files. Please change the paths in these commands to the
appropiate locations. If using clang, use clang++
instead of g++
.
g++ -c filename.cxx -o filename.o -std=gnu++11 -O2 -I{panda3dinclude}
You will need to replace {panda3dinclude}
with the location of the
Panda3D header files. On Linux, this is likely /usr/include/panda3d/
.
On macOS, this will be in /Library/Developer/Panda3D/include/
.
To generate an executable, you can use the following command:
g++ filename.o -o filename -L{panda3dlibs} -lp3framework -lpanda -lpandafx -lpandaexpress -lp3dtoolconfig -lp3dtool -lp3direct
As above, change {panda3dlibs} to point to the Panda3D libraries. On Linux
this will be /usr/lib/panda3d
or /usr/lib/x86_64-linux-gnu/panda3d
,
whereas on macOS it will be /Library/Developer/Panda3D/lib
.
Here is an equivalent SConstruct file, organized for clarity:
pandaInc = '/usr/include/panda3d'
pandaLib = '/usr/lib/panda3d'
Program('filename.cpp',
CCFLAGS=['-fPIC', '-O2', '-std=gnu++11'],
CPPPATH=[pandaInc],
LIBPATH=pandaLib,
LIBS=[
'libp3framework',
'libpanda',
'libpandafx',
'libpandaexpress',
'libp3dtoolconfig',
'libp3dtool',
'libp3direct'])
To run your newly created executable, type:
./filename
If it runs, congratulations! You have successfully compiled your own Panda3D program!